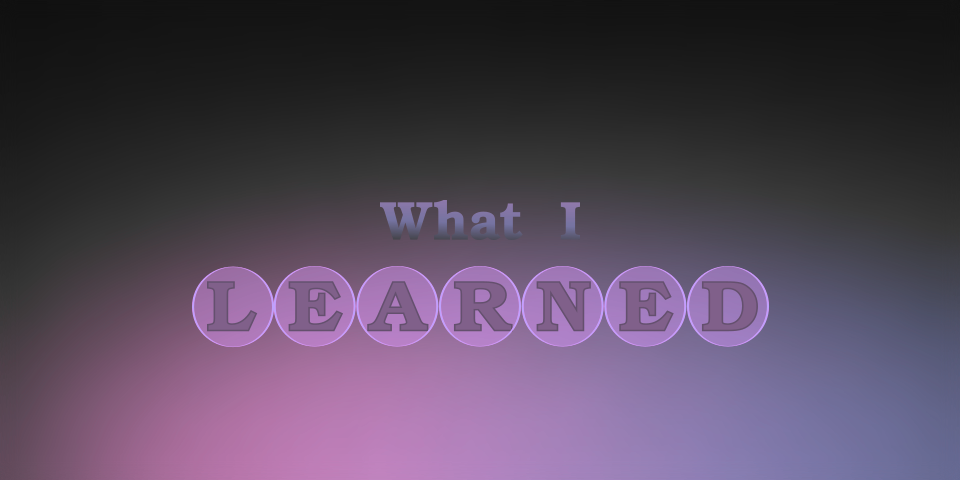
What I learned new this week (week 11)
I am currently working on a flask application, an unsplash like application but with look of a social media application. So most of this post will be about flask.
- In Python Flask, we can set cookies to user device using session. But this cookie will only last for the session.
This is basically Session Storage like cookie storing. To use this you first have to add a secret key to your application.
app.secret_key = 'someVerySecretKey'
. Once you set a secret key just import session from flask and use it as a dictionary.
from flask import Flask, session
app = Flask(__name__)
app.secret_key = 'someVerySecretKey'
@app.route('/set-session')
def set_session():
session['username'] = 'dshaw0004'
session['role'] = 'python developer'
return 'session set'
@app.route('/get-session')
def get_session():
username = session.get('username')
role = session.get('role')
return f'My name is {username} and I am a {role}'
if __name__ == '__main__':
app.run()
- Flask provides a
flash()
function that allows you to store a message in the session that will be available to the next request and rendered on the template. It can be really helpful to show server message to the webpage conditionally. Here is an example of flash -
# server.py
from flask import flash, render_template
@app.route('/')
def index():
flash('some message')
return render_template('index.html')
# index.html
<html><head></head>
<body>
<!-- some other html code -->
{% with flash_message= get_flashed_messages() %}
{% if flash_message %}
<div>
<ul>
{% for message in flash_message %}
<li >{{ message }}</li>
{% endfor %}
</ul>
</div>
{% endif %}
{% endwith %}
</body></html>
-
Python Flask have a thing called BluePrint. It basically let’s you work on a part of your flask application totally separately in a subfolder of your main project directory. And at the end you just have to register the BluePrint on your main flask app. Truly saying I can’t enplain it properly. I want to only one thing “if you use Flask and don’t know about it then try it once”. NeuralNine has a great video on Flask BluePrint, you can watch that.
-
If you have watch NeuralNine’s video on Flask BluePrint and you use the file structure shown on his video then there will be some changes in the
extends & include
. Generally we do{% extends "base.html" %}
but while using BluePrint we have to do{% extends "<foldername>/base.html" %}
, here<foldername>
is the name of the folder insidetemplate
folder on which all the html files are store. Example :{% extends "helloworld/base.html" %}
That’s it for this week. Meeting you next week.